8. Event handling. Module event¶
The event module hanles the events ocurring in the application.
event.py:
def before_event(error, event):
...
def after_event(event):
...
def error_event(event):
...
The events occur on application data changes made by a user
and the API allows to control the events.
The application generates the events by performing
definite actions. These call the corresponding functions
before_event()
, after_event()
or error_event()
from event module.
The events are caused by data changing operations, therefore the general meanings of these functions are the following:
- before_event() - an action before data changing;
- after_event() - an action after data changing;
- error_event() - handling of error on data changing.
8.1. Event generating¶
In the moment a user is changing data, before writing the changes into the database, the before_event()
function is called. If an exception is generated in the body of the function, the writing into the database is cancelled and the user is displayed an exception message.
If the code is executed without any exceptions raised, the changes are committed to the database.
If an error occcurs while committing changes, e.g. the internet connection lost or the user has no permission to make the changes, the error_event()
function is called.
If the changes committed correctly, the after_event()
function is called.
Event without errors:
before_event(event)
...
# the application saves the changes
...
after_event(event)
Event with exception raised in before_event:
before_event(event)
...
raise Exception('Cancel changes')
...
# the application displays an exception message
Event with changes commit error:
before_event(event)
...
# the application saves the changes... Error occured
# the application displays an error message
...
error_event(error, event)
Diagram of the event
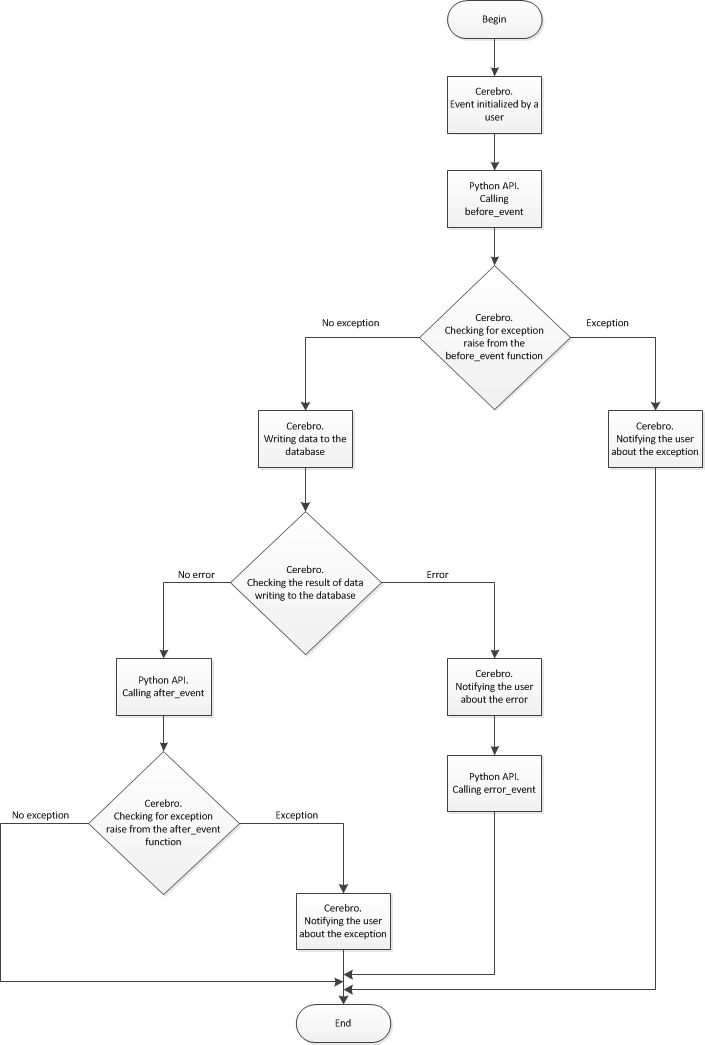
Let’s see how an event occurs, for example, while creating a message in the Cerebro application.
A user writes a message, attaches files there and presses the “Send” button. At this moment the before_event()
function is called.
event.py:
def before_event(event):
...
If an exception is raised while executing the before_event()
function, the user is shown a notification and the new message is voided. Still, the message input form with the text and attachments remains active.
event.py:
def before_event(event):
...
raise Exception('Ouch')
...
If the code is executed without exceptions, the new message is added into the database.
If an error occurs while committing, the message is not added, the user gets a notification with the error cause and the error_event()
function is called.
event.py:
def error_event(error, event):
...
If the message is added successfully, the after_event()
function is called.
event.py:
def after_event(event):
...
If an exception is raised while executing the after_event function, then the user is displayed a message with the exception.
8.2. Event handling functions¶
-
event.
before_event
(event)[source]¶ An event object with defined properties is sent into the function. Object properties allow to define how the data will be changed and to make user-defined changes.
The body of the function may make changes to data and perform different checking routines, passing the results to the application. This is a method to check the data changes made by user before writing these into the database, with an opportunity to correct or cancel them on the way. An exception has to be raised to cancel an event (a data change). It will stop event processing and display a corresponding notification.
If no exception is raised during the function execution, the changes are written into the database, taking possible corrections into account.
-
event.
after_event
(event)[source]¶ An event object, being put into the function, has some definite properties which make it possible to define what kind of changes the data has undergone.
The function is called after writing data into the database. It’s possible, for example, to call API functions changing some additional data, or to launch third-party applications in it.
If an error occurs on this stage you can notify a user about it by raising an exception.
-
event.
error_event
(error, event)[source]¶ Being called, this function gets an error object and an event object which let us determine what kind of error occured and what event was accompanying it. The function is called in case an error occured on writing data to the database.
Event object classes are described in the cerebro.events
module of the cerebro
package.
8.3. Types of events¶
Types of events are differentiated by types of changes to the data. For example, there are types like message creation
, task creation
, progress change
, etc.
There is a set of object description classes for every event type
,
which are put into the event handling functions.
The event object interface
is determined by the type of event and handling function.
event.py:
def before_event(event):
if (event.event_type() == event.EVENT_CREATION_OF_MESSAGE) # creating a message
# event object uses interface of the BeforeEventCreationOfMessage class
def after_event(event):
if (event.event_type() == event.EVENT_CREATION_OF_MESSAGE) # creating a message
# event object uses interface of the AfterEventCreationOfMessage class
def error_event(error, event):
if (event.event_type() == event.EVENT_CREATION_OF_MESSAGE) # creating a message
# event object uses interface of the BeforeEventCreationOfMessage class
As a rule, the before_event()
function gets class objects
,
with names starting with Before…
These objects have the functions that let us know the current values, new values and let us input additional changes.
def before_event(event):
if (event.event_type() == event.EVENT_CHANGING_OF_MESSAGE) # editing a message
omessage = event.original_message() # getting object of original message
if (event.work_time() != omessage.work_time()) # comparing current working time value of the message with the new value input by user.
raise Exception('Working time can not be changed!')
event.set_client_visible(True) # making it visible for clients
The after_event()
function gets class objects
with names starting with After… These objects have the functions that let us know new values.
def after_event(event):
if (event.event_type() == event.EVENT_CHANGING_OF_TASKS_NAME) # changing task name
task_name = event.name() # getting new name
The error_event()
function gets error object
and class objects
which names begin with Before… This is the same object that is input into the before_event()
function but without an opportunity to make changes.
All event types are described in the cerebro.events
module of the cerebro
package in the Event event base class
.
You can find the examples of event handling here.